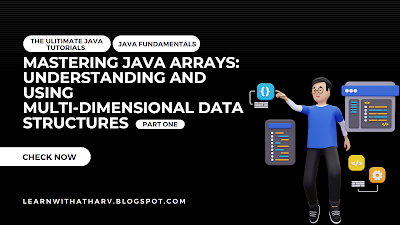 |
MASTERING JAVA ARRAYS |
Arrays in Java provide a convenient way to store and manage collections of data. They are a fundamental building block in Java programming, making it possible to store multiple values of the same data type in a single variable. Whether you're a beginner or an experienced Java developer, understanding how to use arrays effectively is crucial for writing efficient and effective code. In this blog post, we will explore the basics of arrays in Java, including how to declare, initialize, access, and iterate through them. Get ready to take your Java skills to the next level with arrays!
Declaring an Array:
Before you can use an array in Java, you need to declare it. The syntax for declaring an array is as follows:
Here, dataType is the type of data you want to store in the array, and arrayName is the name you choose for the array. For example, to declare an array of integers, you can write:
Initializing an Array:
Once you have declared an array, you can initialize it with a set of values. There are two ways to initialize an array in Java:
1) Initializing an array with a fixed size and values:
int[] num = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
2) Initializing an array with a specific size:
Note that if you initialize an array with a specific size, you need to assign values to each element manually.
Accessing Array Elements
Once you have initialized an array, you can access its elements by using the array name and an index in square brackets. The index of the first element in an array is 0, and the index of the last element is
arrayName.length - 1.
For example, to access the second element in the numbers array, you can write:
int secondElement = num[1];
Iterating Through an Array
To iterate through all the elements of an array, you can use a for loop. Here's an example:
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
In this example,
numbers.length gives you the length of the numbers array, and the for loop runs from 0 to
numbers.length - 1.
Here's a perfect example of how to use arrays in Java:
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5}; // declaring and initializing an array of integers
System.out.println("The array elements are:");
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]); // accessing and printing each element in the array
}
int sum = 0;
for (int i = 0; i < numbers.length; i++) {
sum += numbers[i]; // calculating the sum of all elements in the array
}
System.out.println("The sum of all elements in the array is: " + sum);
}
}
In this example, we declare and initialize an array of integers called
numbers. We then use a
for loop to access each element in the array and print it to the console. Finally, we use another
for loop to calculate the sum of all elements in the array and print the result to the console.
This simple example demonstrates the basic features of arrays in Java, including how to declare, initialize, access, and iterate through them.
In conclusion, arrays are an indispensable tool in Java programming, providing a means of storing and managing collections of data in an organized and efficient manner. Whether you're a beginner or an experienced Java developer, understanding how to use arrays effectively is essential for writing high-quality code. With the knowledge you have gained in this article, you are now equipped to start using arrays in your own Java projects and take your coding skills to the next level!